ThrottleFirst(second = x)
이벤트가 발생하면 첫 이벤트만 통지하고, 이후 x초동안 발생하는 이벤트는 무시한다.
x초 후에는 다시 첫 이벤트가 올때까지 대기한다.
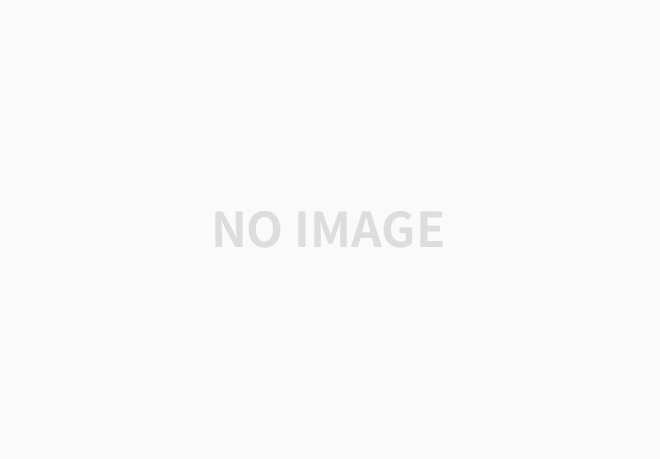
더보기
using System;
using System.Collections;
using UnityEngine;
using UniRx;
public class Game : MonoBehaviour
{
IntReactiveProperty _property = new IntReactiveProperty();
IEnumerator Start()
{
_property
.ThrottleFirst(TimeSpan.FromSeconds(1f))
.Subscribe(value => Debug.Log(value)); // 이벤트 통지: 0 (1초 대기)
yield return new WaitForSeconds(0.5f);
_property.Value = 1; // X
yield return new WaitForSeconds(0.5f);
_property.Value = 2; // 이벤트 통지: 2 (1초 대기)
yield return new WaitForSeconds(1f);
yield return new WaitForSeconds(0.5f);
_property.Value = 3; // 이벤트 통지: 3 (1초 대기)
yield return new WaitForSeconds(0.5f);
_property.Value = 4; // X
yield return new WaitForSeconds(0.5f);
_property.Value = 5; // 이벤트 통지: 5 (1초 대기)
}
}
Throttle(second = x)
이벤트가 발생하면 x초 대기후 통지한다.
대기중 새 이벤트가 발생하면 기존 이벤트는 무시하고 다시 x초를 대기후 새 이벤트를 통지한다.
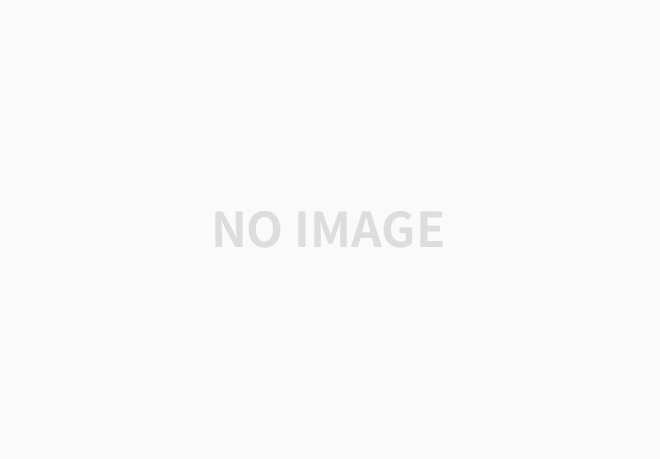
더보기
using System;
using System.Collections;
using UnityEngine;
using UniRx;
public class Game : MonoBehaviour
{
IntReactiveProperty _property = new IntReactiveProperty();
IEnumerator Start()
{
_property
.Throttle(TimeSpan.FromSeconds(1f))
.Subscribe(value => Debug.Log(value)); // 이벤트 발생 (1초 대기)
yield return new WaitForSeconds(1f); // 1초후 이벤트 통지: 0
_property.Value = 1; // 이벤트 발생 (1초 대기)
yield return new WaitForSeconds(0.5f);
_property.Value = 2; // 이벤트 발생 (1초 다시 대기)
yield return new WaitForSeconds(0.5f);
_property.Value = 3; // 이벤트 발생 (1초 다시 대기)
yield return new WaitForSeconds(1f); // 1초후 이벤트 통지: 3
}
}
ThrottleFrame(frame = 1)
이벤트가 발생하면 1프레임 대기후 마지막으로 발생한 이벤트만 통지한다.
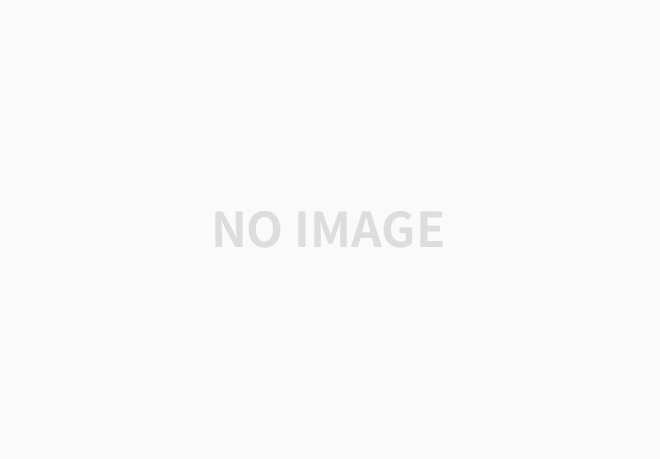
더보기
using System.Collections;
using UnityEngine;
using UniRx;
public class Game : MonoBehaviour
{
IntReactiveProperty _property = new IntReactiveProperty();
IEnumerator Start()
{
_property
.ThrottleFrame(1)
.Subscribe(value => Debug.Log(value));
_property.Value = 1;
_property.Value = 2;
yield return null; // 1프레임후 이벤트 통지: 2
}
}